Ludum Dare is an online “game jam” and competition where participants from all over the world make a game of their own from scratch in 48 hours or less (or 72 hours, for the more informal version that goes on at the same time). There are some basic rules, a theme to work with, and everyone just goes at it. It takes place a few different times over the course of the year, and I honestly didn’t plan to take part at all.
But then the theme was announced to be “minimalism”. Early on, as their interpretation of the theme most people seemed to using the simplest graphics possible. But I began to wonder what other possibilities there were and started reading up on the minimalism movement. While most people are familiar with it as an art movement, it also covered various other forms of design, most notably architecture. In architecture, minimalism wasn’t just about making things as simple as possible, it was also about finding multiple visual and functional uses for a single element in the design. I was already stewing on jumping in and trying the event out, but learning about this was where inspiration really struck.
I decided that I was going to make a mini-RPG where all the graphics came from a single 128×128 pixel sprite sheet divided into 8×8 pixel tiles, and only a palette of 16 colors were allowed. Oh, and the 16 colors had to all be 8-bit (3 bits each for red and green, and then 2 for blue). I was looking forward to this!
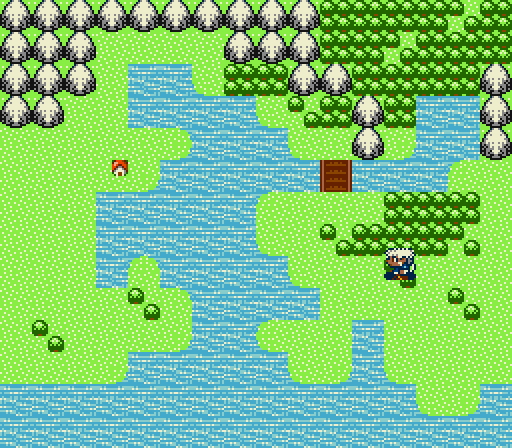
A single 8×8 tile can be flipped and mirrored to make all the corners of the coastline.
Of course, an RPG is a bit of a daunting task to finish in just 48 hours. There’s an overworld, dungeons, towns, shops, battle screens, enemies, status menus—all sorts of elements that have to come together to make an adventure. But who said I’d need all of these things? Minimalism was the theme, after all, and the less there was in the game the less I’d have to find room for in the cramped sprite sheet. I’d just shave the game down to the bare essentials. A tiny handful of enemies to fight, twelve or so screens worth of simple map to explore, and a basic menu system. Even battles can be streamlined to the bare essentials. Many people do RPGs for Ludum Dare, it’s not like the idea is unusual. So, Saturday morning, I was all ahead full.
But come Monday evening—the end of the relaxed 72 hour version of the challenge—I just barely had the thing playable. It was my own fault for using a game engine that wasn’t quite as complete as I thought it was, but even as the deadline neared I could still have pulled it off by greatly reducing the scope of the game to just the six or seven screens of map that were finished and tapping “fight” to automatically win battles. That’s minimalism, right? It counts. With a few lines of dialog here and there it could have even been a fun ten minute game.
But I didn’t want to do that.
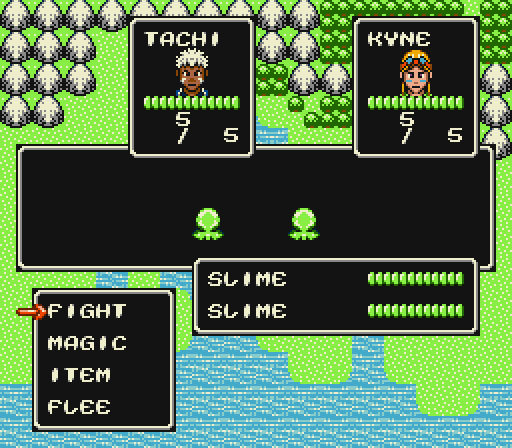
These slimes are small, leaving more sprite room for larger enemies elsewhere.
No, I’d fallen too madly in love with the idea. Just how much game could I cram into those handful of 8×8 pixel tiles? The font alone took up almost a quarter of the space on the sprite sheet, so whenever I want to add something, I have to jumble things around, find things that can be taken out, find things that can be reused elsewhere in different ways. And yet I’ve already got a world map with forests and deserts and mountain ranges. I’ve got caves and forest paths and castles to explore. I’ve got not just one character, but a little party that fights together as a team. Even with these few things to work with, I think I could make an engaging “full” game.
I’m tempted to put Junction aside for awhile and just keep running with it. With a month or so of work, I’d probably have a pretty cool little game that’d be worth a dollar or two. I think I’ll toy with it a little longer before committing to it, or putting it aside until later.